View the Data Logging – FAQ for quick resolution to common data logging issues.
To resolve database engine login error view the Database Security Login topic.
Remote OAS Service Access
The OAS Service must be started to be accessed by a local or remote OPC client component or application. Use the OAS Service Control application to Start the Service.
If you are using a Firewall make sure to open up TCP port 58727 for both inbound and outbound traffic. You can find what port number is used under Configure-Options-Networking in the service, 58727 is the current default port number.
You can check if the TCP port is open using the Windows Command Prompt with the following command on the same computer as the OAS Service.
netstat -an | find “58727”
If the service is not started or the port is not open then the command will just return without any results.
To check a remote computer port use PortQry from Microsoft.
Download PortQry from Microsoft.
Run PortQryV2.exe to extract to desired directory.
Use the Windows Command Prompt from the PortQryV2 directory with the following command. Replace 127.0.0.1 with the IP address of the remote system where the OAS Engine is running.
portqry -n 127.0.0.1 -e 58727
If this test fails check the network connection to the remote system and open the TCP port for incoming and outbound traffic in the firewall and antivirus software.
Videos – Develop for iOS and Android
View the following video for a quick introduction to the developing native interfaces for iOS and Android applications.
PCL HMI
Create iOS and Android Native Apps for live data read and write access.
PCL for iOS and Android Applications
NOTE: The OASPCL assembly still ships with the OAS Platform installation for legacy support, but we have now made the OASData and OASConfig assemblies compatible with Xamarin projects. More information on how to use these assemblies can be found here:
OASData – real time data access
OASConfig – programmatic platform configuration
The video below details how to develop native apps for iOS and Android using the PCL component. Using the OASData and OASConfig components follow the same method. Just replace the OASPCL with the OASData for reading and writing real time tag data, and OASConfig for configuring OAS servers.
How to create HMI applications for live data for iOS and Android applications.
Getting Started – .NET Core for iOS and Android
NOTE: The OASPCL assembly still ships with the OAS Platform installation for legacy support, but we have now made the OASData and OASConfig assemblies compatible with Xamarin projects. More information on how to use these assemblies can be found here:
OASData – real time data access
OASConfig – programmatic platform configuration
Prerequisites:
- Windows Development for iOS and Android apps
- Visual Studio 2012 or newer (2017 or 2019 Recommended) for Windows
- Xamarin Android Player or Android Emulator (if building Android Apps)
- Separate machine or Virtual Machine with Mac OS X and XCode (if building iOS Apps)*
— or —
- Mac Development for iOS and Android apps
- Visual Studio for Mac (download)
- Deployment
- An active subscription to the Apple Developer Program for iOS distribution via the App Store
- An active Google Developer account for Android deployment via the Google Play Store
*Note: when developing for iOS with Visual Studio for Windows, you will need to have access to a Mac on your network that has XCode installed. Debugging and execution on the iOS Simulator actually runs on the connected Mac and not on the PC hosting Visual Studio.
For more information about Xamarin Requirements for iOS development on Windows see the following: https://developer.xamarin.com/guides/cross-platform/getting_started/requirements/#windows
Step 1 – Install Xamarin Forms or VS for Mac
The instructions below are for Visual Studio 2015. Read more on Installation of Xamarin Forms for all versions of Visual Studio including VS 2017 for Windows.
See the following for Installation of Visual Studio for Mac.
Step 2 – Create a New Visual Studio project
Create a new project in Visual Studio. If Xamarin is installed properly, this will be under
Templates > Visual C# > Cross-Platform.
Select the project type of Blank App (Xamarin.Forms Shared). This will create a single shared application code base, and individual projects for each deployment target, including Android, iOS, Windows Universal Platform, Windows Phone and Windows.
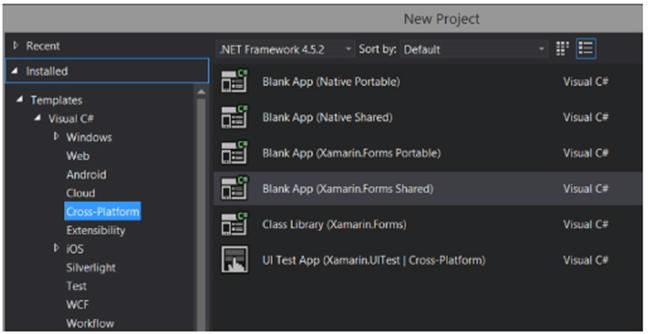
For this project we’ll only be using the Android and iOS projects, so once the solution is created, remove all other project targets so you’re left with just the shared app code and the projects for the the desired targets.
After clearing out the extra projects, your solution should look like this:
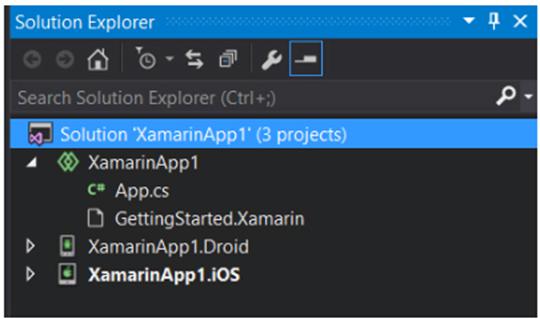
Step 3 – Add OAS Component References
Add references to the OAS assemblies. These are located in your Open Automation Software installation directory (typically C:\Program Files\Open Automation Software\OAS).
You need to add references to the following files in every individual deployment target project. So in this case, you need to add these references to both the iOS and Android projects.
OASData.dll
OASConfig.dll
These assemblies are the .NET Core Open Automation Software components for reading and writing Tag data and for configuring OAS Platform settings.
Step 4 – Code: add component instances
Update shared source code in the App.cs file. This will house all of the application code and will be shared by both iOS and Android projects to make it easier to maintain code for multiple devices.
First, add in a reference to the OASPCL namespace, along with application-level variables.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using OASData; using OASConfig; using Xamarin.Forms; namespace XamarinApp1 { public class App : Application { OASConfig opc = new OASConfig(); OASData opcd = new OASData(); string server = "10.211.55.3"; // your machine IP bool bPumpSet = false; bool bPumpVal = false; Label lblSine = new Label { Text = "Loading...", HorizontalTextAlignment = TextAlignment.Center }; Label lblPump = new Label { Text = "Loading...", HorizontalTextAlignment = TextAlignment.Center }; Button btnPump = new Button { Text = "Toggle Pump" };
The OASConfig component is used to configure OAS Server and tag settings, and is not used in this example, but the OASData component is.
This is used to monitor tags, fire events when values change on the server, and also to update values on the server. These are the local variables used:
- Server – A string that holds a reference to the IP address of your OAS server where we will be reading and writing data.
This may be the localhost of the machine with Visual Studio, but the compiled mobile apps may not be running locally, so localhost or 127.0.0.1 may not work.
This needs to be the IP address on the network for your OAS server. - bPumpSet – A boolean flag that determines if we have gotten values from the server for the Pump.Value tag so we know when it’s safe to allow updates.
- PumpVal – A boolean to hold the current value for the Pump.Value tag.
- lblSine – A Xamarin Forms label to display the value of the Sine.Value tag
- lblPump – A Xamarin Forms lable to display the value of the Pump.Value tag
- btnPump – A Xamarin Forms button used to toggle the value of Pump.Value
Step 5 – Code: handle events
Now we’ll add some code to monitor server tags and handle updates when values change. The AddTag method adds a tag to the list of tags to monitor. You can also use the AddTags method to add more than one at a time.
The ValuesChangedAll event is fired every time the server values have changed for any tag being monitored. This event will be passed a list of tags, values, data qualities, and timestamps for each update. If values have not changed for some tags, they will not be included in the arrays passed to the event.
protected override void OnStart () { // set up tags opcd.AddTag(string.Format(@"\\{0}\Sine.Value", server)); opcd.AddTag(string.Format(@"\\{0}\Pump.Value", server)); // set up event handler opcd.ValuesChangedAll += Opcd_ValuesChangedAll; } private void Opcd_ValuesChangedAll(string[] Tags, object[] Values, bool[] Qualities, DateTime[] TimeStamps) { // iterate thru received values and update display Device.BeginInvokeOnMainThread(() => { int idx = 0; foreach (string t in Tags) { if (t.Contains("Sine.Value") && Values[idx] != null) { lblSine.Text = Values[idx].ToString(); } if (t.Contains("Pump.Value") && Values[idx] != null) { bPumpSet = true; bPumpVal = (bool)Values[idx]; lblPump.Text = bPumpVal.ToString(); } idx++; } }); }
In the event handler, we will iterate through the list of tags and update labels when their respective values have changed.
Note: We must execute UI updates within a Lambda (a C# anonymous function) tied to the Device.BeginInvokeOnMainThread. This hands over the execution to the main UI thread. If this is not done, crashes or unexpected results could occur.
Step 6 – Code: update screen
The last bit of code will be to handle the clicking of a button and triggering an update on the server when that happens. First we’ll update the App constructor to include the new labels and button in the layout. Then we add a Clicked handler to the button.
public App () { // The root page of your application MainPage = new ContentPage { Content = new StackLayout { VerticalOptions = LayoutOptions.Center, Children = { lblSine, lblPump, btnPump } } }; btnPump.Clicked += BtnPump_Clicked; } private void BtnPump_Clicked(object sender, EventArgs e) { // change pump value here if (bPumpSet) { Device.BeginInvokeOnMainThread(() => { opcd.SyncWriteTags(new string[] { string.Format(@"\\{0}\Pump.Value", server) }, new object[] { !bPumpVal }); }); } }
We use the SyncWriteTags method to send up a list of tag/value pairs. In this case we’re just sending up the Pump.Value tag and the inverse of the current value to effectively toggle it.
Step 7 – Building and testing for Android
Start by running the Xamarin Android Player. If you do not have this installed, you can download it here. You’ll then need to download and install device images that the Player will use. For this example, we chose the Nexus 7 with Marshmallow build of the Android OS.
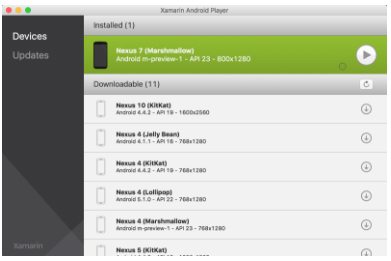
Once you’ve downloaded the device image you can start it up by hitting the Play button. This will run the device emulator. After the device has started, you can then click on the gear icon to get details. The important field is the IP address. We’ll use this to connect Visual Studio for debugging.
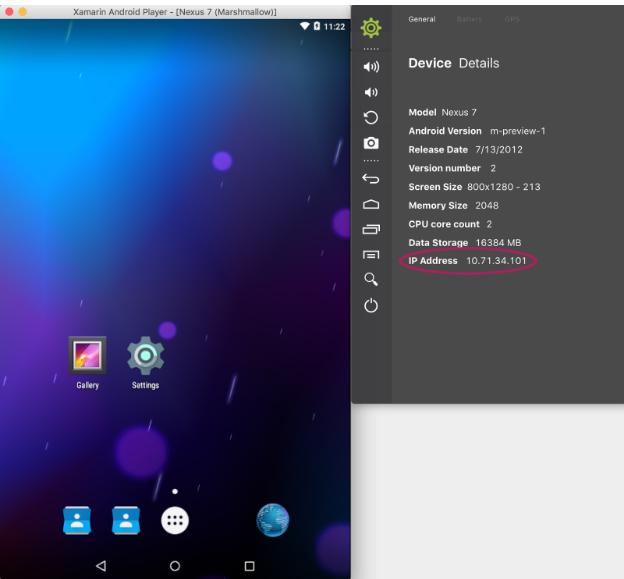
Now, you can go back to Visual Studio, select the Android Project, to be sure we’ll be debugging that one and not the iOS version. Select Debug to ensure we are in Debug and not Release mode. Then go to the set of icons in the toolbar and select Open Android Adb Command Prompt:
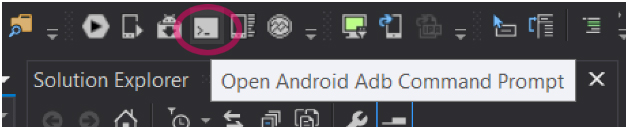
When the command prompt opens, enter the following command:
adb connect 10.71.34.101
Replace the IP above with the IP of your Android device.
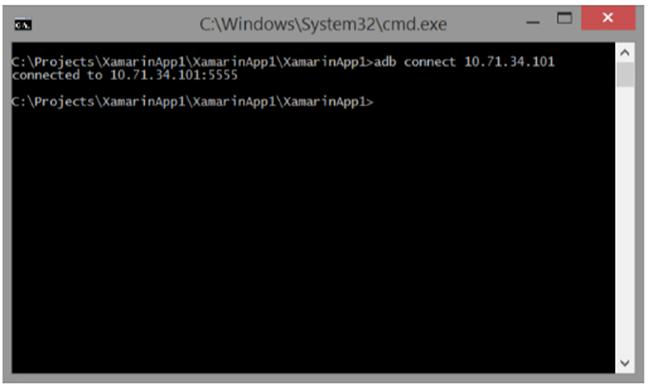
You should see a successful connection. You’re now ready to debug the project.
In the Visual Studio toolbar, make sure your Android Device is selected as the target and then select Start Debugging. The project will build and then be deployed to the device.
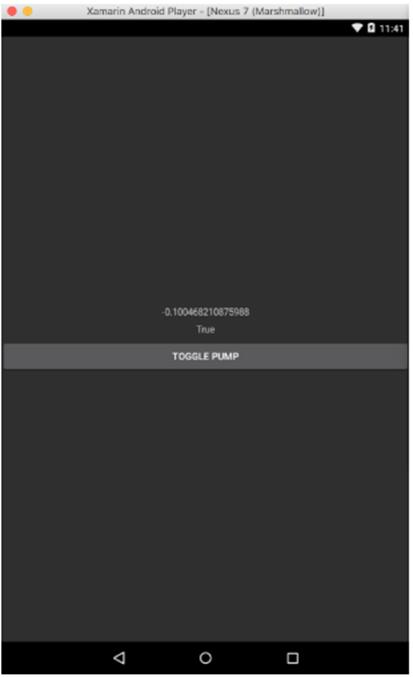
Note: If you get an error related to missing “provisioning profiles”, this is common. To fix it, select the iOS project, then be sure to select the iPhoneSimulator as the target. Then return to the Android project and try again. Even though we were debugging the Android project, the iOS project will compile and throw errors.
Step 8 – Building and testing for iOS
As stated earlier, to build and test an iOS app, you must be connected to a Mac on your network. This Mac must have XCode installed along with the Xamarin Mac Agent. This allows Visual Studio to connect to the Mac and use its Simulator for debugging.
The simplest way to get the Xamarin Mac Agent on your test machine is to install Xamarin Studio for the Mac found at http://www.xamarin.com/download.
Once you have your Mac configured and accessible on the network, return to Visual Studio to connect by selecting Xamarin Mac Agent from the toolbar:
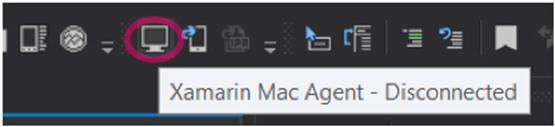
Locate your Mac in the list and click Connect. If it is not on the list, click Add Mac… to enter connection details manually.
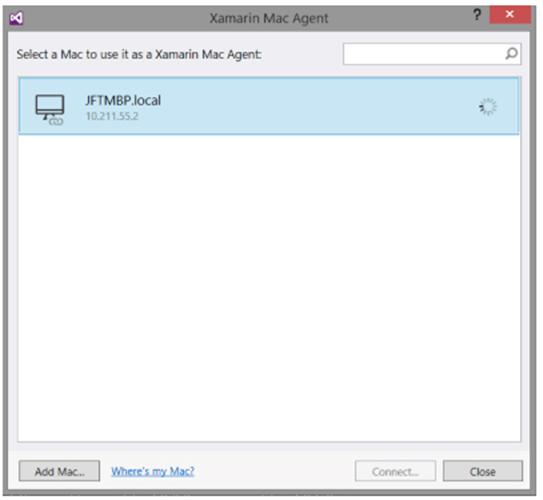
Once connected, the toolbar icon will change and the link icon will appear in the Xamarin Mac Agent dialog:
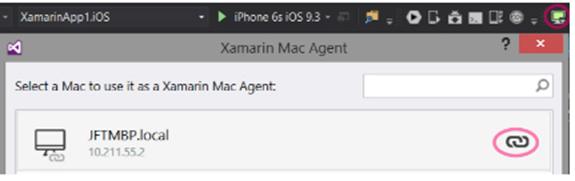
You can now select the iOS project and target the iPhoneSimulator, choosing the model you want to test. In this case we’ve chosen the iPhone 6s. The models and iOS versions available to you will depend on the version of XCode and Simulator installed on your Mac.

Now select Start Debugging and the app will compile, deploy to the simulator and start. Switch over to your Mac and you should see it running.
As you can see, the labels and button are similar to those on the Android version, but particular to the iOS app. This allows you to develop an app that is familiar to each target platform user while remaining on a single code base.
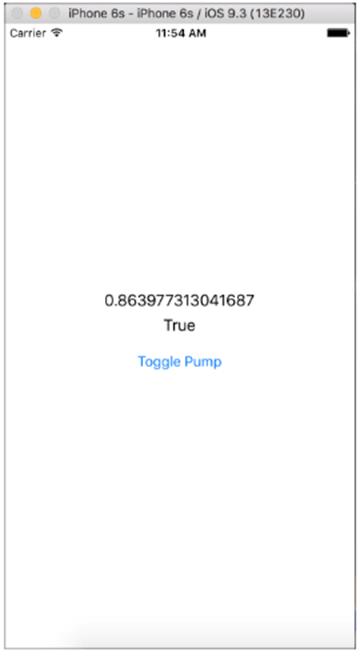
Resources
For more details on how to use the OPCSystemsComponent or the OPCSystemsData components, you can refer to the Programmatic Interface section of our online help. The PCL versions of these components have the same interface as the standard .NET components, so all code examples will function in the same way.
Xamarin Tools and Support
https://www.xamarin.com
Xamarin Android Player
https://www.xamarin.com/android-player
Additional Demo Applications
OAS Tanks HMI for iOS and Android
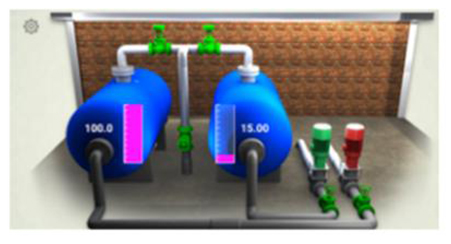
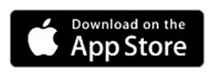
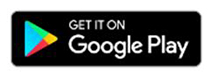
Overview – .NET Core for iOS and Android
Build iOS, Android, Windows Phone and Universal Windows Platform (UWP) apps in a familiar .NET environment – all with a single shared code base
Cross-platform development with .NET Core 2.x
The OAS Platform ships with two components built on the .NET Core 2.0 Framework. The OASData and OASConfig components allow you to read and write real time Tag data, and programmatically configure OAS servers. With these components, you can write a single code base and use it to deploy apps for multiple platforms, including native iOS and Android apps using Visual Studio and Xamarin extensions.
What’s the advantage of using .NET Core?
Before .NET Core, there was no easy way to share a single code base between applications targeting the various desktop, mobile, and device platforms. You were forced to create and maintain a code base and project for each.
Now, with .NET Core you can create and maintain a single project and build a single assembly that can be shared between IDEs and platforms. You can even use Visual Studio, one of the best IDEs for application development.
Are apps developed fully native?
Yes, apps developed using Xamarin within Visual Studio are compiled to fully native apps for the target platform.
You can develop HMI apps for iOS, Android, Windows Phone, and the Universal Windows Platform (UWP) all from a single solution containing shared code along with additional code for each target.
NOTE: The OASPCL assembly still ships with the OAS Platform installation for legacy support, but we have now made the OASData and OASConfig assemblies compatible with Xamarin projects. More information on how to use these assemblies can be found here:
Getting Started – Tags
Tags are used to define data source communications, alarm limits, and other real-time signal properties like Time On and Counts for keeping track how long a point has been on and how many times it has transitioned in a given period. Tags are common data sources to all clients. Data Sources can be setup to communicate with Modbus, Allen Bradley, Siemens controllers, MQTT, and OPC Servers.
To use data directly from a Visual Studio application use the default data source of Value as a fixed value that can be changed using the OPC Controls Data component. Tags can also be used to setup Calculations from other local and remote Tags.
The following section is how to manually add and define Tags using the Configure OAS application. Tags can also be added and modified using the CSV Import and Export selections using the Configure-Tags application to use Excel or other third party Comma Separated Variable editor.
To learn how to programmatically add or modify Tags from your own Visual Studio application refer to the following article on how to add and define multiple tags with one method:
OAS System Config – Get and Set Tag Properties
For a complete list of all Tag properties refer to the OAS Configuration – Tags section in this help file./sdk/articles/oasconfig04.html
Please view the Getting Started with Open Automation Software Video to familiarize yourself with installation, setting up Tags, selecting Data Sources and Destinations, and implementing Networking and Security.
Step 1
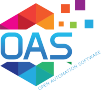
Start Configure OAS application from the program group Open Automation Software.
Step 2
Select Configure-Tags.
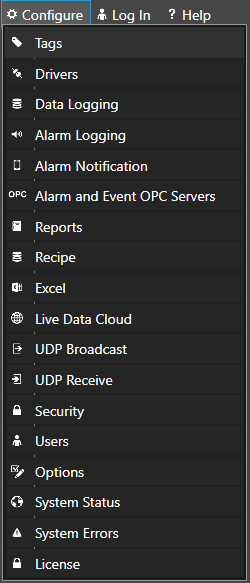
Step 3
Select the Local OAS Service by selecting the Select button.

Note: The Configure application can be used to connect to remote systems using the network node name or IP address of the remote node the OAS Service is running on. Simply enter the IP Address or network node name of the remote OAS Service you wish to connect to and click on the Select key.
Note: When selecting a service if you receive a warning dialog that the service cannot be retrieved make sure the OAS Service, OAS Data Service, and OAS Database Service are started as described in Start Service.
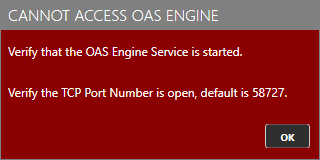
Step 4
If you already have the default Demo Tags loaded you can right in the list of tags to the right to Delete All Tags.
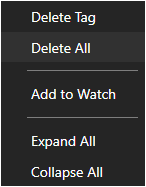
Click on the Add Tag button located at the top of the Tag browser.
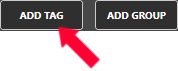
Note
You can also add organizational Groups as many levels deep as you prefer and add tags to groups. To do this first add a Group to the root level, then right click on the Group in the right window to add additional Groups or Tags.
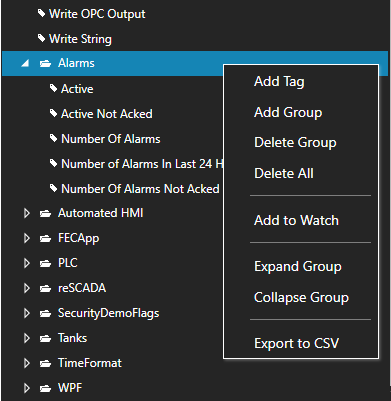
Step 5
Enter the Tag name Ramp in the Add Tag dialog box.
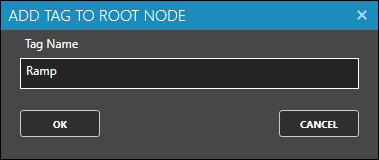
Step 6
Repeat Steps 4 and 5 with Tag name Sine.
Step 7
Repeat Steps 4 and 5 with Tag name Random.
Step 8
Select Tag Ramp in the right Tag window.
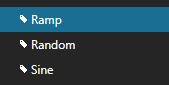
All Tag properties will appear in the lower window.
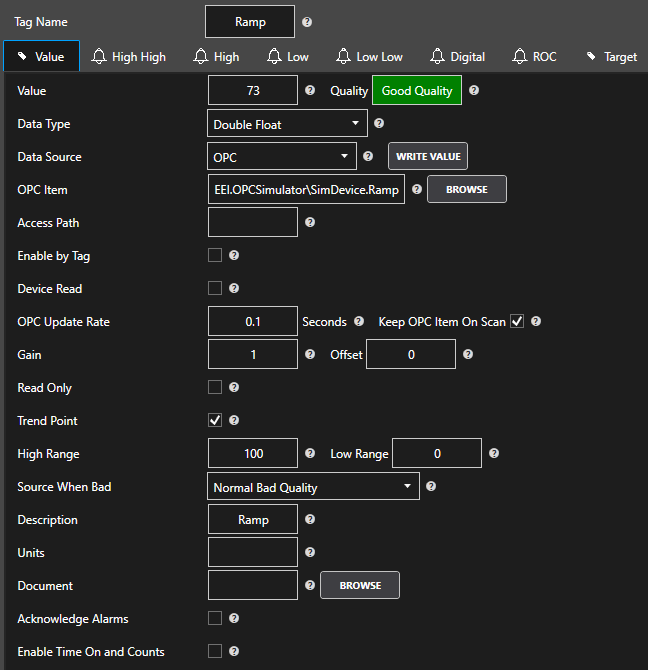
Step 9
For the Value Parameter set the Data Source to OPC Item.
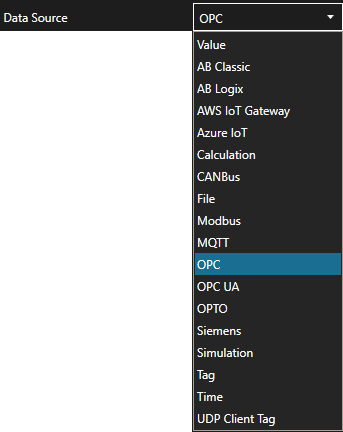
Step 10
Use the OPC Browse button at the right of the OPC Item to browse OPC Servers.

Step 11
Expand Local to expand EEI.OPCSimulator and select SimDevice.
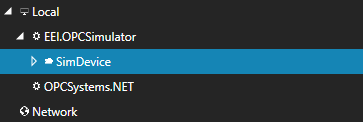
Step 12
Select Ramp from the list of OPC Items and select OK to enter the OPC Item EEI.OPCSimulator\SimDevice.Ramp.
Step 13
If you plan to use trending on this point enable the Trend Point option in the upper right of the Tag Properties window.

Step 14
Set the Description field to Ramp.
Step 15
Select the High High Parameter and set the Value field to 80 and enable the High High alarm.
Step 16
Select the High Parameter and set the Value field to 60 and enable the High alarm.
Step 17
Select the Low Parameter and set the Value field to 40 and enable the Low alarm.
Step 18
Select the Low Low Parameter and set the Value field to 20 and enable the Low Low alarm.
Step 19
Select the Apply Changes button in the lower right corner.

Step 20
Select Tag Random and the Value Parameter.
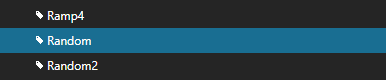
Repeat Steps 8 through 14 and Step 19 substituting Ramp for Random as Tag name and OPC Item name.
EEI.OPCSimulator\SimDevice.Random.
Step 21
Select Tag Sine and the Value Parameter.

Repeat Steps 9 through 19 substituting Ramp for Sine as Tag name and OPC Item name. Use 0.9 for High High Value, 0.8 for High Value, 0.2 for Low Value, and 0.1 for Low Low Value.
EEI.OPCSimulator\SimDevice.Sine.
Step 22
Select the Save button on the toolbar at the top.

Step 23
Create a directory on the local C:\ drive with the name OASDemo.
Save the file DemoTags.tags in the directory C:\OASDemo.
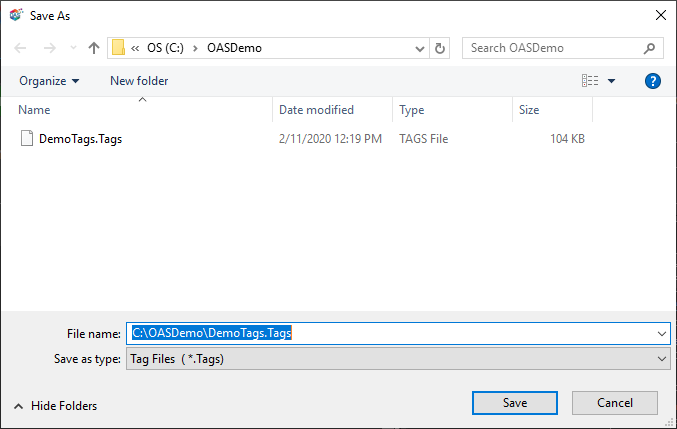
Step 24
Under Configure – Options set the Default Tag Configuration File so when the computer restarts the tag file will automatically be loaded.
Getting Started Azure IoT Data Hub
View the following video for a complete demonstration of how to send live data to Azure IoT Data Hub.
- 0:00 – Introduction
- 0:17 – Create an IoT Hub
- 0:47 – Step 1 Azure Portal
- 0:55 – Step 2 Create a Resource
- 1:25 – Step 3 Project Details
- 1:31 – Step 4 Resource Group
- 1:45 – Step 5 IoT Hub Name
- 1:54 – Step 6 Review Create
- 2:03 – Step 7 Deployment is Underway
- 2:21 – Step 8 Overview
- 3:18 – Step 9 Shared Access Policies
- 3:25 – Create an IoT Driver
- 4:25 – Publish Tags
- 6:15 – Monitor Values with VS Code
- 6:38 – Conclusion
Create an IoT Hub
You need to create an IoT Hub for your device to connect to. The following steps show you how to complete this task using the Azure portal:
Step 1
Sign in to the Azure portal.
Step 2
From the left menu, click Create a resource, then click Internet of Things, and then click IoT Hub.
Step 3
On the Basic tab, enter your Project Details.
Step 4
In Resource Group, create a new resource group, or select an existing one. For more information, see Using resource groups to manage your Azure resources.
Step 5
In the IoT Hub Name field, enter a name for your hub. If the Name is valid and available, a green check mark appears in the Name box.
Step 6
When you have finished entering your IoT hub configuration options, click Review + Create at the bottom of the page. On the next page, review your details and then click Create.
Step 7
A page will appear that says: Your deployment is underway.
It can take a few minutes for Azure to create your IoT hub. Be patient.
When it is done you will see a page that says: Your deployment is complete. Click the Go to resource button.
Step 8
The page you now see gives you an Overview of your new IoT Hub. On the top right side of the page, you will see the Hostname for your hub. Copy this somewhere because you will need it later on in the tutorial to set up your OAS driver.
Step 9
Next click Shared access policies from the left menu. In the pane that appears to the right, click iothubowner.
In the panel that appears to the right, select the Copy to clipboard icon next to Connection string – primary key. Save this as well, you will need it later.
You have now created your IoT hub and you have the hostname and connection string you need to complete the rest of this tutorial.
Create an IoT Driver
Step 1
Open Configure UDI.
Step 2
Select Configure >> Drivers from the top menu.
Step 3
Select your Network Node, either local or remote.
Step 4
Enter the Driver Interface Name you wish to use.
Step 5
Select Azure IoT from the Driver combo box.
Step 6
Enter the Azure IoT Device ID you want to use.
Step 7
Enter the Connection String from Step 9 of the previous section into the Azure IoT Connection field.
Step 8
Enter the hostname from Step 8 of the previous section into the Azure IoT Hub URL field.
Step 9
To enable data buffering when communication failure occurs check Enable Store and Forward. Values will be stored in the directory specified under Configure >> Options >> Store and Forward.
Step 10
Select the preferred Azure IoT Transport. Typically it is AMQP.
Optionally define a secondary failover IoT Hub URL if the primary server fails with the property Enable Failover.
If both the primary and secondary servers are offline the Return to Online settings determines the retry frequency.
View Driver Interface Failover for more information and and video demonstrating communications failover.
Step 11
Click Add Driver on the top left.
Publish Live Data to your Azure IoT Hub.
There are 2 ways to publish data from OAS to Azure IoT Hub. Both require Tags to be setup first for the data sources you want to transfer.
Option 1 – Publish Selected Tags to your Azure IoT Hub.
View the following video for a complete demonstration of how to publish data to Azure IoT Data Hub.
- 00:00 – Introduction
- 00:23 – Set up Tags in OAS
- 00:41 – Configure Azure IoT
- 01:53 – Publish Selected Tags
- 05:31 – Visual Studio Code
- 08:00 – Step by step instructions
- 08:12 – Bulk Publish to AWS IOT
- 09:21 – Step by step instructions / Publish Data to AWS IOT Gateway
- 09:33 – Bulk Publish to mqtt broker
- 10:29 – MQTT Explorer
- 11:20 – Step by step instructions/ Getting Started MQTT
- 11:34 – Save button of the OAS Configuration tool
Step 1
Enable Publish Selected Tags at the bottom of the Driver configuration.
Step 2
Select to publish data continuously at a specified interval, based on event, or at a specific time of day.
If Event Driven browse for a local or remote OAS tag that will trigger the publish. Select a Boolean tag that will change state from false to true, true to false, or both. Or choose an Integer tag that trigger a publish anytime the value changes other than 0.
Step 3
Enable Publish Latest Value Only to send only the latest value of each tag when published or disable to send all value changes since the last time a publish occurred.
Enabled Include All Tags Each Publish to send at least the latest value of each tag when published or disable to only send the tags that have changed since the last publish.
Enable Publish All Tags As One Topic to publish all tag values as one topic or disable to send each tag as its own topic.
See examples in Step 6 below for each selectable option.
Step 4
Specify the Publish Topic Id if choosing to Publish All Tags As One Topic.
Specify the Tag Id, Value Id, an optional Quality Id, and Timestamp Id for each tag value that is sent.
When including the Timestamp Id also specify the timestamp format, use Custom to specify your own date and time format.
Step 5
Specify local and remote OAS tag variables to include in each publish and specify the Id. Value is the current value from the data source or you can select any of the over 600 tag variables of each tag to publish.
Optionally use CSV Export and CSV Import buttons to set up additional tags to publish using Microsoft Excel.
When selecting remote tags use Basic Networking syntax or Live Data Cloud syntax in the tag path.
Step 6
Select Apply Changes to begin publishing to Azure IoT Hub. Select Save to save the new driver configuration within the tag file.
Examples:
Examples of publishing every 2 seconds with each tag value changing every second:
{
{
{
Option 2 – Use Data Route to Send Data to Azure IoT Hub
Step 1
Select Configure >> Tags from the top menu.
Step 2
Select your Network Node, either local or remote.
Step 2
From the demo tags select the Ramp Tag.
Step 3
Select the Target tab.
Step 4
Enable Write to target.
Step 5
Select the Azure IoT in the Target Type dropdown.
Step 6
Select the Driver interface you created.
Step 7
Apply the Changes and you should now be writing to your IoT Hub.
Step 8
The message is formatted as follows.
{“deviceId”:”myFirstDevice”,”TagName”:”Ramp”,”Value”:66,”DataType”:”DoubleFloat”,”Quality”:true,”TimeStamp”:”2016-04-11T14:38:53.7125255″}
.
Getting Started – WPF HMI Dashboard
- A ready to go template with built in trending and alarming.
- Template for developing new windows.
- Designed to run on multiple PC’s that have different screen resolutions.
- Provides 10 user customizable desktop displays based on user login.
- User selections are persisted across all PC terminals.
- Demonstrates the use of Open Automation Software Custom Objects.
- Demonstrates how to theme an application.
- Demonstrates the use and development of gadgets.
- Demonstrates the use of styles in WPF.
- A learning tool for developing your own WPF applications.
- A Window for displaying Reports
- A Window for displaying XPS documents
- To download the HMI Dashboard Project go to Download Source Code Examples.
- Once you have unzipped it you can open it with Visual Studio or Expression Blend.
Videos – WPF Page Navigation
FAQs – Software Licensing
- A warning appears when you use the Configure application.
- Also the Runtime will not start if you have too many Open Automation Software Tags loaded.
- If you are using DirectOPC once you reach the tag limit no other DirectOPC Items will be added.
- You can see how many tags you are using under Configure-System Status in the Totals section.
- If you upgrade your software to the latest version without an active maintenance plan that covers that version.
- If you change the CPU mother board. This includes cloning the image of the drive and restoring to a new PC.
- If you remove all of the hard disks from the system that were present when the software was licensed.
- With older OAS version 9 or less if you remove all of the Ethernet cards from the system that were present when the software was licensed.