The following is a list of markup attributes that can be added to any HTML element. Each attribute adds a behavior, tied to one or more OAS server Tags. You can combine attributes to generate the behavior you desire. If you prefer more control over behavior and would like to write your own logic, see the Client Script Library Reference.
Text
- valid config fields:
- formats
<div id="txt_ex1" oas-tag-txt='{"tag":"Sine.Value"}'></div>
<div id="txt_ex2" oas-tag-txt='{"tag" : "Sine.Value", "config" : {"formats" : { "bad_q":"????", "string" : "Sine = {0}"} } }'></div>
<div id="txt_ex3" oas-tag-txt='{"tag" : "Sine.Value", "config" : {"formats" : { "bad_q":"????", "string":"Sine = {0}", "float":"0.000"} } }'></div>
Value :
- valid config fields:
- formats
<input id="val_ex1" type="text" oas-tag-val='{"tag":"Sine.Value"}'/>
Background:
<button id="bkg_ex1" oas-tag-bkg='{ "type": "group", "all_f": { "color": "#f00" }, "bad_q": { "color": "#fc0" }, "group": [ { "tag": "RandBool.Value", "config": {"color": "#0f0"} } ] }'>BUTTON</button>
Foreground:
<button id="fg_ex1" oas-tag-fg='{ "type": "group", "all_f": { "color": "#fc0" }, "bad_q": { "color": "#f00" }, "group": [ { "tag": "RandBool.Value", "config": { "color": "#fff"} } ] }'>BUTTON</button>
<button id="fg_ex2" oas-tag-bkg='{ "type": "group", "all_f": {"color": "#f00"}, "bad_q": {"color": "#fc0"}, "group": [ { "tag": "RandBool.Value", "config": {"color": "#0f0"} } ] }' oas-tag-fg='{ "type": "group", "all_f": {"color": "#fc0"}, "bad_q": {"color": "#f00"}, "group": [ { "tag": "RandBool.Value", "config": { "color": "#fff"} } ] }'>BUTTON</button>
Border :
<button id="brd_ex1" style="border-width: 2px;" oas-tag-brd='{ "type": "group", "all_f": {"color": "#fc0"}, "bad_q": {"color": "#f00"}, "group": [ { "tag": "RandBool.Value", "config": { "color": "#fff"} } ] }'>BUTTON</button>
<button id="brd_ex2" style="border-width: 2px;" oas-tag-bkg='{ "type": "group", "all_f": {"color": "#f00"}, "bad_q": {"color": "#fc0"}, "group": [ { "tag": "RandBool.Value", "config": {"color": "#0f0"} } ] }' oas-tag-fg='{ "type": "group", "all_f": {"color": "#fc0"}, "bad_q": {"color": "#f00"}, "group": [ { "tag": "RandBool.Value", "config": { "color": "#fff"} } ] }' oas-tag-brd='{ "type": "group", "all_f": {"color": "#fc0"}, "bad_q": {"color": "#f00"}, "group": [ { "tag": "RandBool.Value", "config": { "color": "#fff"} } ] }'>BUTTON</button>
Image Source :
- config:
- relative or absolute URL of an image
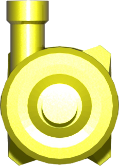
<img id="src_ex1" style="width: 55px;" src="https://openautomationsoftware.com/wp-content/uploads/2022/05/Pump-Yellow-Front.png" oas-tag-src='{ "type": "group", "all_f": "https://openautomationsoftware.com/wp-content/uploads/2022/05/Pump-Red-Front.png", "bad_q": "https://openautomationsoftware.com/wp-content/uploads/2022/05/Pump-Yellow-Front.png", "group": [ { "tag": "RandBool.Value", "config": "https://openautomationsoftware.com/wp-content/uploads/2022/05/Pump-Green-Front.png" } ] }'/>
Background Flash :
<button id="bkg_fl" style="border-width: 2px;" oas-tag-bkg-fl='{ "tag": "RandBool.Value", "config": { "color": "#f00", "trigger": "on_true" } }'>BUTTON</button>
Foreground Flash :
<button id="fg_fl" style="border-width: 2px;" oas-tag-fg-fl='{ "tag": "RandBool.Value", "config": { "color": "#f00", "trigger": "on_true" } }'>BUTTON</button>
Border Flash :
<button id="brd_fl" style="border-width: 2px;" oas-tag-brd-fl='{ "tag": "RandBool.Value", "config": { "color": "#f00", "trigger": "on_true" } }'>BUTTON</button>
Tooltip :
- config:
- formats
NOTE:Not all browsers will display the tooltip, but the title attribute of the element will be set and could still be used for screen readers or other applications
<button id="tt_ex" style="border-width: 2px;" oas-tag-tt='{"tag": "Sine.Value"}'>BUTTON</button>
Enable :
<button id="en_ex" oas-tag-txt='{ "tag": "RandBool.Value", "config": { "formats": { "bool_f": "Enabled", "bool_t": "Disabled" } } }' oas-tag-en='{ "tag": "RandBool.Value", "config": { "trigger": "on_false" } }'>BUTTON</button>
Visible :
<button id="vis_ex" oas-tag-vis='{ "tag": "RandBool.Value", "config": { "bad_q" : false, "trigger": "on_true" } }'>BUTTON</button>
Opacity :
<button id="op_ex" oas-tag-op='{ "tag": "Ramp2.Value", "config": { "formats" : { "bad_q" : 0.25 }, "gain" : 2.0 } }'>BUTTON</button>
Width :
<button id="wd_ex" oas-tag-wd='{ "tag": "Random.Value", "config": { "formats" : { "bad_q" : 200 }, "gain" : 1.0, "offset" : 100 } }'>BUTTON</button>
Height :
<button id="ht_ex" oas-tag-ht='{ "tag": "Random.Value", "config": { "formats" : { "bad_q" : 50 }, "gain" : 1.0, "offset" : 50 } }'>BUTTON</button>
Scale-X :
<button id="sx_ex" oas-tag-sx='{ "tag": "Ramp2.Value", "config": { "formats" : { "bad_q" : 1.0 }, "gain" : 5.0 } }'>BUTTON</button>
Scale-Y :
<button id="sy_ex" oas-tag-sy='{ "tag": "Ramp2.Value", "config": { "formats" : { "bad_q" : 1.0 }, "gain" : 5.0 } }'>BUTTON</button>
Translate-X :
<div id="tx_ex" style='width:15px;height:15px;background-color:#c33;' oas-tag-tx='{ "tag":"Ramp2.Value", "config":{ "formats":{ "bad_q":10 }, "gain":100, "offset":10 } }'></div>
Translate-Y :
<div id="tx_ex" style='width:15px;height:15px;background-color:#c33;' oas-tag-ty='{ "tag":"Ramp2.Value", "config":{ "formats":{ "bad_q":10 }, "gain":100, "offset":10 } }'></div>
Set :
example 1 – simple boolean toggle:
{ "tag":"Pump.Value", "config":{ "evt":"click", "set":"toggle" } }
example 2 – toggle with confirmation dialog:
{ "tag" : "Pump.Value", "config" : { "evt" : "click", "set" : "toggle", "set_confirm" : true } }
example 3 – user input:
{ "tag" : "Pump.Value", "config" : { "evt" : "click", "set" : "input" } }
example 4 – customized input dialog:
{ "tag" : "Pump.Value", "config" : { "evt" : "click", "set" : "input", "set_confirm_msg" : "Please enter a value that will be set for the Pump.Value server tag.", "set_confirm_title" : "Set Pump.Value" } }
example 5 – using a value from another field. Use the set_src for the value to be submitted for the tag. This should be the element id holding the new value:
{ "tag" : "Pump.Value", "config" : { "evt" : "click", "set" : "value", "set_src" : "element_id" } }